src¶
Submodules¶
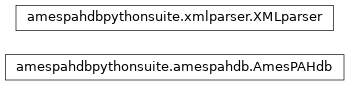


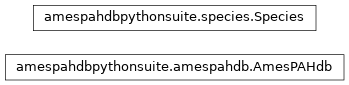




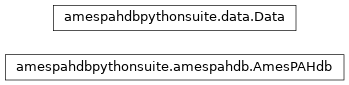










amespahdb class¶
- class amespahdbpythonsuite.amespahdb.AmesPAHdb(**keywords)[source]¶
Bases:
object
AmesPAHdbPythonSuite main class. Contains methods to parse the database, perform search based on query, and retrieve UIDs. Calls classes:
amespahdbpythonsuite.transitions.Transitions
,amespahdbpythonsuite.laboratory.Laboratory
,amespahdbpythonsuite.species.Species
,amespahdbpythonsuite.geometry.Geometry
, to retrieve the respective instances.- checkversion(version: str) bool [source]¶
Method to check against a PAHdb version.
- Returns:
Boolean whether a provided version matched the PAHdb version.
- getdatabaseref() dict [source]¶
Method to retrieve the database.
- Returns:
Dictionary containing the parsed database.
- getgeometrybyuid(uids: list[int] | int) Geometry [source]¶
Retrieve and return geometry instance based on UIDs input. UIDs should be a list, e.g. the output of search method. Calls the
amespahdbpythonsuite.geometry.Geometry
class andamespahdbpythonsuite.amespahdb.__getkeybyuids()
method.- Parameters:
- uidslist of integers
List of UIDs.
- Returns:
geometry instance
- getlaboratorybyuid(uids: list[int] | int) Laboratory | None [source]¶
Retrieve and return laboratory database instance based on UIDs input. UIDs should be a list, e.g. the output of search method. Calls the
amespahdbpythonsuite.laboratory.Laboratory
class.- Parameters:
- uidslist of integers
List of UIDs.
- Returns:
laboratory database instance
- getspeciesbyuid(uids: list[int] | int) Species [source]¶
Retrieve and return species instance based on UIDs input. UIDs should be a list, e.g. the output of search method. Calls the
amespahdbpythonsuite.species.Species
class.- Parameters:
- uidslist of integers
List of UIDs.
- Returns:
species instance
- gettransitionsbyuid(uids: list[int] | int) Transitions [source]¶
Retrieve and return transitions instance based on UIDs input. UIDs should be a list, e.g. the output of search method. Calls the
amespahdbpythonsuite.transitions.Transitions
class.- Parameters:
- uidslist of integers
List of UIDs.
- Returns:
transitions instance
- static message(text, space: int = 55) None [source]¶
A method to print terminal message.
- Parameters:
- textstring or list of strings.
Text to be displayed.
- spaceinteger
Number to indent the text.
data class¶
- class amespahdbpythonsuite.data.Data(d: dict | None = None, **keywords)[source]¶
Bases:
object
AmesPAHdbPythonSuite data class
- difference(uids: list[int]) None [source]¶
Updates data to the difference with provided UIDs.
- Parameters:
- uidslist of integers
List of UIDs.
xmlparser class¶
xmlparser.py
Parse a NASA Ames PAH IR Spectroscopic Database XML library file, with or without schema checking.
- class amespahdbpythonsuite.xmlparser.XMLparser(filename: str | None = None, validate: bool = False)[source]¶
Bases:
object
Parse a NASA Ames PAH IR Spectroscopic library XML-file.
Optional behavior includes validating against a schema.
- Attributes:
filename (str): XML filename. validate (bool): Whether to validate the XML schema or not. library (dict): Dictionary containing the parsed data.
- Examples:
parser = XMLparser(filename=”xml_file.xml”) parser.verify_schema() dict = parser.to_pahdb_dict()
parser = XMLparser(filename=”xml_file.xml”, validate=True) dict = parser.to_pahdb_dict()
parser = XMLparser() parser.filename = “xml_file.xml” parser.validate = True dict = parser.to_pahdb_dict()
- to_pahdb_dict(validate: bool = False) dict [source]¶
Parses the XML, with or without validation.
- Args:
validate (bool). Defaults to self.valdiate value, but can be overridden.
- Note:
Sets the attribute self.library when successful.
- Returns: library (dict): Dictionary, with the UIDs as keys,
containing the transitions, geometry data, as well as UID metadata, references, comments, and laboratory.
transitions class¶
- class amespahdbpythonsuite.transitions.Transitions(d: dict | None = None, **keywords)[source]¶
Bases:
Data
AmesPAHdbPythonSuite transitions class. Contains methods to create and apply an emission model.
- static absorption_cross_section(f: ndarray) ndarray [source]¶
Calculates the PAH absorption cross-section per Li & Draine 2007, ApJ, 657:810-837.
- Params f:
frequencies in wavenumber
- Returns:
float array
- static approximate_attained_temperature(T: float) float [source]¶
Calculate a PAH’s temperature after absorbing a given amount of energy using an approximation.
- Parameters:
T (float) – Excitation temperature in Kelvin.
- static approximate_feature_strength(T: float)[source]¶
Calculate a feature’s strength convolved with a blackbody using an approximation from Bakes, Tielens & Bauschliher, ApJ, 556:501-514, 2001.
: Param T: Excitation temperature in Kelvin.
- static attained_temperature(T: float) float [source]¶
Calculate a PAH’s temperature after absorbing a given amount of energy.
- Parameters:
T (float) – Excitation temperature in Kelvin.
- calculated_temperature(e: float | dict | None, **keywords) None [source]¶
Applies the Calculated Temperature emission model.
- Parameters:
e (float) – Excitation energy in erg or temperature in Kelvin.
- cascade(e: float, **keywords) None [source]¶
Applies the Cascade emission model.
- Parameters:
e – Excitation energy in erg.
- Type:
float
- convolve(**keywords) Spectrum [source]¶
Convolve transitions with a line profile. Calls class:
amespahdbpythonsuite.spectrum.Spectrum
to retrieve the respective instance.
- static feature_strength(T: float) float [source]¶
Calculate a feature’s strength covolved with a blackbody.
- Parameters:
T (float) – Excitation temperature in Kelvin.
- fixed_temperature(t: float) None [source]¶
Applies the Fixed Temperature emission model.
- Parameters:
t (float) – Excitation temperature in Kelvin.
- get() dict [source]¶
Calls class:
amespahdbpythonsuite.data.Data.get
to get keywords.
- static heat_capacity(T: float) float [source]¶
Calculate heat capacity.
- Parameters:
T (float) – Excitation temperature in Kelvin.
- static isrf(f: ndarray) ndarray | float [source]¶
Callback function to calculate the absorption cross-section times the interstellar radiation field per Mathis et al. 1983, A&A, 128:212.
- Params f:
frequencies in wavenumber
- Returns:
float array
- static isrf_approximate_feature_strength_convolved(f: ndarray) ndarray [source]¶
Calculate a feature’s strength convolved with the interstellar radiation field using an approximation.
- Param f:
frequencies in wavenumber
- Returns:
float
- static isrf_feature_strength_convolved(f: ndarray) ndarray [source]¶
Calculate a feature’s strength convolved with the interstellar radiation field.
- Param f:
frequencies in wavenumber
- Returns:
float
- static isrf_number_of_photons(f: ndarray) ndarray | float [source]¶
Callback function to calculate the number of photons per Mathis et al. 1983, A&A, 128:212.
- Params f:
frequencies in wavenumber
- Returns:
float array
- static isrf_squared(f: ndarray) ndarray | float [source]¶
Callback function to calculate the absorption cross-section times the interstellar radiation field per Mathis et al. 1983, A&A, 128:212.
- Params f:
frequencies in wavenumber
- Returns:
float array
- static mean_energy(**keywords)[source]¶
Callback function to calculate the mean energy in erg for a given blackbody temperature.
- Returns:
float array
- static mean_energy_squared(**keywords)[source]¶
Callback function to calculate the mean energy in erg^2 for a given blackbody temperatyre.
- Returns:
float array
- static number_of_photons(**keywords)[source]¶
Calculate the number of photons given a blacbody radiation field.
- static planck(f: ndarray) ndarray [source]¶
Callback function to Calculate the absorption cross-section multiplied by Planck’s function.
- Params f:
frequencies in wavenumber
- Returns:
float array
- static planck_approximate_feature_strength_convolved(f: ndarray) ndarray [source]¶
Calculate a feature’s strength convolved with a given blackbody using an approximation.
- Param f:
frequencies in wavenumber
- Returns:
float
- static planck_feature_strength_convolved(f: ndarray) ndarray [source]¶
Calculate a feature’s strength convolved with a given blackbody radiation field.
- Param f:
frequencies in wavenumber
- Returns:
float
- static planck_number_of_photons(f: ndarray) ndarray [source]¶
Callback function to Calculate the number of photons using Planck’s function.
- Params f:
frequencies in wavenumber
- Returns:
float array
- static planck_squared(f: ndarray) ndarray [source]¶
Callback function to Calculate the absorption cross-section multiplied by Planck’s function squared.
- Params f:
frequencies in wavenumber
- Returns:
float array
- set(d: dict | None = None, **keywords) None [source]¶
Calls class:
amespahdbpythonsuite.data.Data.set
to parse keywords. Checks flavor of the database, i.e., theoretical or experimental
- static stellar_model_approximate_feature_strength_convolved(f: ndarray) ndarray [source]¶
Calculate a feature’s strength convolved with the interstellar radiation field using an approximation.
- Param f:
frequencies in wavenumber
- Returns:
float
- amespahdbpythonsuite.transitions.Tstar: float¶
- amespahdbpythonsuite.transitions.charge: int¶
- amespahdbpythonsuite.transitions.energy: float | dict | ndarray | None¶
- amespahdbpythonsuite.transitions.frequencies: ndarray¶
- amespahdbpythonsuite.transitions.frequency: float¶
- amespahdbpythonsuite.transitions.intensities: ndarray¶
- amespahdbpythonsuite.transitions.nc: int¶
- amespahdbpythonsuite.transitions.star_model: Any¶
spectrum class¶
- class amespahdbpythonsuite.spectrum.Spectrum(d: dict | None = None, **keywords)[source]¶
Bases:
Transitions
AmesPAHdbPythonSuite spectrum class. Contains methods to fit and plot the input spectrum.
- coadd(weights: dict = {}, average: bool = False) Coadded [source]¶
Co-add PAHdb spectra.
- Parameters:
- weights: dict
Dictionary of fit weights to use when co-adding.
- average: bool
If True calculates the average coadded spectrum.
- fit(y: Observation | Spectrum1D | list, yerr: list = [], notice: bool = True, **keywords) Fitted | None [source]¶
Fits the input spectrum.
- get() dict [source]¶
Calls class:
amespahdbpythonsuite.transitions.Transitions.get
. Assigns class variables from inherited dictionary.
- mcfit(y: Observation | Spectrum1D | list, yerr: list = [], samples: int = 1024, uniform: bool = False, multiprocessing: bool = False, notice: bool = True, **keywords) MCFitted | None [source]¶
Monte Carlo sampling and fitting to the input spectrum.
Parameters¶
- samplesNumber of samples.
int
- set(d: dict | None = None, **keywords) None [source]¶
Calls class:
amespahdbpythonsuite.transitions.Transitions.set
to parse keywords.
coadd class¶
- class amespahdbpythonsuite.coadded.Coadded(d: dict | None = None, **keywords)[source]¶
Bases:
Spectrum
AmesPAHdbPythonSuite coadded class
- get() dict [source]¶
Calls class:
amespahdbpythonsuite.spectrum.Spectrum.get
. Assigns class variables from inherited dictionary.
species class¶
- class amespahdbpythonsuite.species.Species(d: dict | None = None, **keywords)[source]¶
Bases:
object
AmesPAHdbPythonSuite species class. Contains methods to work with PAH species.
- difference(uids: list[int]) None [source]¶
Updates data to the difference with provided UIDs.
- Parameters:
- uidslist of integers
List of UIDs.
- geometry() Geometry [source]¶
Return geometry instance. Calls the
amespahdbpythonsuite.geometry.Geometry
class.- Returns:
geometry instance
- intersect(uids: list[int]) None [source]¶
Updates data to the intersection with provided UIDs.
- Parameters:
uids : list of integers
- laboratory() Laboratory | None [source]¶
Return laboratory instance. Calls the
amespahdbpythonsuite.laboratory.Laboratory
class.- Returns:
laboratory instance
- transitions() Transitions [source]¶
Return transitions instance. Calls the
amespahdbpythonsuite.transitions.Transitions
class.- Returns:
transitions instance
geometry class¶
- class amespahdbpythonsuite.geometry.Geometry(d: dict | None = None, **keywords)[source]¶
Bases:
Data
AmesPAHdbPythonSuite geometry class
- bec() dict [source]¶
Convert PAH carbon and hydrogen positions to a boundary-edge code. Only makes sense to use this for regular PAHs. Return of a tuple with a list of boundary carbons traversed in order and the boundary-edge code. By Dr. Joseph E. Roser <Joseph.E.Roser@nasa.gov>
- diagonalize(full: bool = False, equal: bool = False) None [source]¶
Diagonalize the moment of inertia and align structure with the x-y plane
- set(d: dict | None = None, **keywords) None [source]¶
Calls class:
amespahdbpythonsuite.data.Data.set
to parse keywords.
laboratory class¶
- class amespahdbpythonsuite.laboratory.Laboratory(d: dict | None = None, **keywords)[source]¶
Bases:
Data
AmesPAHdbPythonSuite laboratory class. Contains methods to work with a laboratory spectrum.
- set(d: dict | None = None, **keywords) None [source]¶
Calls class:
amespahdbpythonsuite.data.Data.set
to parse keywords.
fitted class¶
- class amespahdbpythonsuite.fitted.Fitted(d: dict | None = None, **keywords)[source]¶
Bases:
Spectrum
AmesPAHdbPythonSuite fitted class
- get() dict [source]¶
Calls class:
amespahdbpythonsuite.spectrum.Spectrum.get
. Assigns class variables from inherited dictionary.
- getclasses(**keywords) dict | None [source]¶
Retrieves the spectra of the different classes of the fitted PAHs.
- geterror() dict | None [source]¶
Calculates the PAHdb fitting uncertainty as the ratio of the residual over the total spectrum area.
- getsizedistribution(nbins: int = 0, min: float | None = None, max: float | None = None) tuple [source]¶
Retrieves the size distribution of the fitted PAHs.
- set(d: dict | None = None, **keywords) None [source]¶
Calls class:
amespahdbpythonsuite.spectrum.Spectrum.set
to parse keywords.
mcfitted class¶
- class amespahdbpythonsuite.mcfitted.MCFitted(d: dict | None = None, **keywords)[source]¶
AmesPAHdbPythonSuite mcfitted class. Contains methods to fit and plot the input spectrum using a Monte Carlo approach.
- get() dict [source]¶
Calls class:
amespahdbpythonsuite.transitions.Transitions.get
. Assigns class variables from inherited dictionary.
observation class¶
- class amespahdbpythonsuite.observation.Observation(d: None = None, **keywords)[source]¶
Bases:
object
AmesPAHdbPythonSuite observation class. Contains methods to work with astronomical spectra.
- read(filename: Path | str) None [source]¶
Read a spectrum.
- Parameters:
- filename: str
Name of file to read.
- rebin(x: ndarray | float, uniform=False, resolution=False) None [source]¶
Resample the spectral data.
Module contents¶
The AmesPAHdbPythonSuite is developed by the NASA Ames PAH IR Spectroscopic Database team, asscociated with the Astrophysics & Astrochemistry Laboratory at NASA Ames Research Center. The NASA Ames PAH IR Spectroscopic Database and pypahdb are being supported through a directed Work Package at NASA Ames titled: “Laboratory Astrophysics - The NASA Ames PAH IR Spectroscopic Database”.
Additional information can be found at the NASA Ames PAH IR Spectroscopic Database website, which is located at https://www.astrochemistry.org/pahdb You are kindly asked to consider the following references for citation when using the AmesPAHdbPythonSuite:
C.W. Bauschlicher, Jr., A. Ricca, C. Boersma, and L.J. Allamandola, “THE NASA AMES PAH IR SPECTROSCOPIC DATABASE: COMPUTATIONAL VERSION 3.00 WITH UPDATED CONTENT AND THE INTRODUCTION OF MULTIPLE SCALING FACTORS”, The Astrophysical Journal Supplement Series, 234, 32, 2018 https://doi.org/10.3847/1538-4365/aaa019
C. Boersma, C.W. Bauschlicher, Jr., A. Ricca, A.L. Mattioda, J. Cami, E. Peeters, F. Sanchez de Armas, G. Puerta Saborido, D.M. Hudgins, and L.J. Allamandola, “THE NASA AMES PAH IR SPECTROSCOPIC DATABASE VERSION 2.00: UPDATED CONTENT, WEBSITE AND ON/OFFLINE TOOLS”, The Astrophysical Journal Supplement Series, 211, 8, 2014 https://doi.org/10.1088/0067-0049/211/1/8
Mattioda, A. L., Hudgins, D. M., Boersma, C., Ricca, A., Peeters, E., Cami, J., Sanchez de Armas, F., Puerta Saborido, G., Bauschlicher, C. W., J., and Allamandola, L. J. “THE NASA AMES PAH IR SPECTROSCOPIC DATABASE: THE LABORATORY SPECTRA”, The Astrophysical Journal Supplement Series, 251, 22, 2020, https://doi.org/10.3847/1538-4365/abc2c8